An easy start!
I can start by saying: There is more to JavaScript then just JavaScript. Scripts written in CRM 4.0, you would use crmForm.all.(...) With CRM 2011, you would use Xrm.Page.(...) Those are (as far as I know!) JavaScript objects or libraries built to make your life easier. When it comes to JavaScript as a whole though, there is jQuery, Dojo, Sencha, Prototype and countless other libraries out there. I recommend not to get hung up worrying you're not catching everything. So for now, I'm just going to focus writing a basic javascript - by starting like this, you'll soon learn that it it is not that complicated!
Writing a script in CRM 2011
A basic script is to look at the value of an Option Set and toggle a section based on the value. When the Relationship Type is set to Customer, a section called “Policy Info” will appear. When the Relationship Type is set to Prospect, the “Policy Info” section will hide again. The purpose for this is to only show to the user what is important for the contact. For example, nn the case of a Prospect, they don’t have a policy, so it doesn’t provide any value to the CRM users.
1. The form we will use to customize
Getting Prepared
To start, we need to add three different schema names: the field, the tab and the section. One way to get the schema names is to click the “Customize” tab and select “Form”. For those who didn't know - this is an excellent way when doing a fast change to the form!2. A quick way to the form customizer!
Once the Form Editor opens up, select the field you want to use (in this case we’re using a custom field). Once you’ve clicked the field, click on the “Change Properties” button on the ribbon at the top of the screen. A new window should pop-up. Click on the Details tab, to see the “Name”. This is the schema name.
3.For javascript purposes, the schema name (shown below as "name") is the value you most often will use (this is also the schema name in a database table). The display name, is as descriped the label end users will see (and is not unique).
You will also need the name for the table. To do this, click just to the right of the text. A light blue border should now be on the tab. At this point, it’s selected so let’s click the “Change Properties” button again.
4. Double clicking should work too ;)
For tabs and sections, the Display tab contains the schema name we need.
Note: If you have a GUID for the name, feel free to rename it to something recognizable.
Next, get the name of the section we are hiding. Be clear you have the following names;
- Relationship Type – customertypecode
- General Tab – general
- Section – Policy
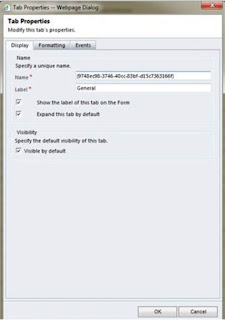
Retreiving the code
Getting a Field
To do this, you’ll want to call:Xrm.Page.getAttribute(“CRM_Field_SchemaName”)So for our example, I’m going to use the “Relationship Type” attribute called customertypecode. To get this attribute, write the following to call:
Xrm.Page.getAttribute(“customertypecode”)
Getting the Fields Value
Now that you have the field, let’s get the value:Xrm.Page.getAttribute(“customertypecode”).getValue()
Writing text between the script
When writing a javascript, try to get used to commenting the script in between the lines. This is both helpful for you and whoever that may edit later. A comment in JavaScript can be done with the double slash “//”.// function togglePolicyInfo(){
// if policy info equals Customer
// show section
// else
// hide section
// }
Inserted
For this post. I'll just skip to a complete script for now, which will look like this: You will see values we retrieved earlier inscripted.function togglePolicyInfo(){
if (Xrm.Page.getAttribute('customertypecode').getValue() == 100000000){
// Customer - show policy information
Xrm.Page.ui.tabs.get("general").sections.get("sectionPolicy").setVisible(true);
} else {
// hide policy information
Xrm.Page.ui.tabs.get("general").sections.get("sectionPolicy").setVisible(false);
}
}